XSLT processor
Learning project: PHP
Educom’s training program introduced me to not only CSS as a styling language but also to Extensible Stylesheet Language (XSL), which is the styling language for XML documents.
What’s the program about?
In reference to that, I worked on a project using the XSLT language to transform a document into an XML document via XSL formatting.
As you can see below in the code section, the start point of this project was the file library.xml. In the file simple.xslt the formatting is being described and the xslt_processor.php converts library.xml file into a new XML format which can be displayed in the browser. The end result you can see in the picture.
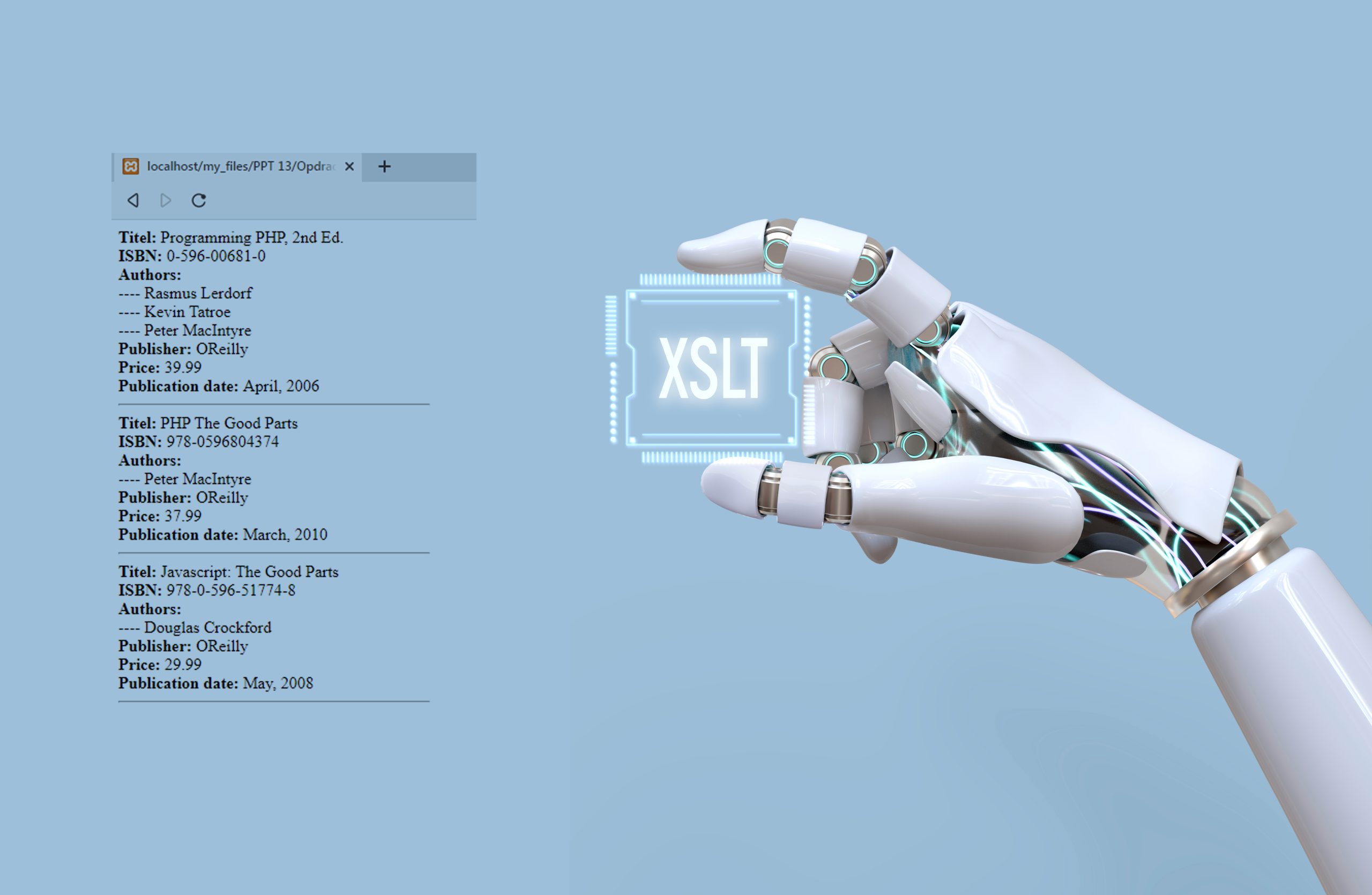
library.xml
simple.xslt
ISBN:
Authors:
Price:
Publication date:
xslt_processor.php